Algorithme de tri par insertion en Java avec exemple de programme
Qu'est-ce que l'algorithme de tri par insertion ?
Le tri par insertion est un algorithme de tri simple adapté aux petits ensembles de données. A chaque itération, l'algorithme :
- Supprime un élément d'un tableau.
- La compare à la plus grande valeur du tableau.
- Déplace l'élément à son emplacement correct.
Processus d'algorithme de tri par insertion
Voici comment fonctionne graphiquement le processus de l'algorithme de tri par insertion :
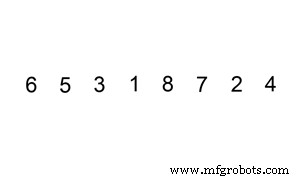
Processus d'algorithme de tri par insertion
Exemple de programme Java pour trier un tableau à l'aide de l'algorithme de tri par insertion :
package com.guru99; public class InsertionSortExample { public static void main(String a[]) { int[] myArray = {860,8,200,9}; System.out.println("Before Insertion Sort"); printArray(myArray); insertionSort(myArray);//sorting array using insertion sort System.out.println("After Insertion Sort"); printArray(myArray); } public static void insertionSort(int arr[]) { int n = arr.length; for (int i = 1; i < n; i++) { System.out.println("Sort Pass Number "+(i)); int key = arr[i]; int j = i-1; while ( (j > -1) && ( arr [j] > key ) ) { System.out.println("Comparing "+ key + " and " + arr [j]); arr [j+1] = arr [j]; j--; } arr[j+1] = key; System.out.println("Swapping Elements: New Array After Swap"); printArray(arr); } } static void printArray(int[] array){ for(int i=0; i < array.length; i++) { System.out.print(array[i] + " "); } System.out.println(); } }
Sortie du code :
Before Insertion Sort 860 8 200 9 Sort Pass Number 1 Comparing 8 and 860 Swapping Elements: New Array After Swap 8 860 200 9 Sort Pass Number 2 Comparing 200 and 860 Swapping Elements: New Array After Swap 8 200 860 9 Sort Pass Number 3 Comparing 9 and 860 Comparing 9 and 200 Swapping Elements: New Array After Swap 8 9 200 860 After Insertion Sort 8 9 200 860
Java
- Fonction calloc() dans la bibliothèque C avec l'EXEMPLE de programme
- Java Hello World :comment écrire votre premier programme Java avec un exemple
- Méthode String Length () en Java:comment trouver avec l'exemple
- Méthode Java String charAt() avec exemple
- Méthode Java String contains() | Vérifier la sous-chaîne avec l'exemple
- Méthode Java String endsWith () avec exemple
- Java BufferedReader :comment lire un fichier en Java avec un exemple
- Algorithme de tri à bulles en Java :programme de tri de tableaux et exemple
- Tri de sélection dans le programme Java avec exemple